Using JavaScript in Viedoc
- Introduction
- Addressing Viedoc items
- Specifying the event
- Specifying the form
- Specifying the item
- Relative path
- Example of using the optional indexer
- Cross event date
- Operators
- Data types
- Pass by value vs. pass by reference
- Passed by value
- Pass by reference
- System variables
- Statistics variables
- Form sequence numbers
- Expressions
- JavaScript expression editor
- Autocomplete and lookup
- Error highlighting
- Links to eLearning
- Unsupported expression types
- Boolean expressions
- Date comparison
- Checking if two dates are the same
- Finding the earliest/latest date
- File properties
- Default value
- Viedoc provided functions
- Array.contains function
- Range item specific functions and properties
- Math library
- Function in item settings
- Debugging your expression
- Validation
- Examples/Use cases
Introduction
In Viedoc Designer, JavaScript can be used to provide a lot more flexibility when working with the study design, by:
- setting Visibility conditions (for items, item groups, activities and events).
- writing data checks under the Validation tab for items.
- using functions/default values for items.
- writing conditions for Alerts.
Important! The syntax used is the JavaScript syntax, as described in ECMAScript 5.1 standards. This is why it is important to verify the functionality against the ECMAScript 5.1 specification and out of range values.
Note! If an incorrectly formatted JavaScript code is put in the study design, the outcome of the executed JavaScript code in Viedoc Clinic will be handled as a "false result" for Visibility conditions, Data checks and Alerts. If used in a Function for an item, the item will not be populated, and a note will show up in the Form Audit record mentioning an issue in the study design.
Note! If the full reference path for an item in an alert body, for example, COMMON_AE.AESPID
is used, and the event is specified in the reference, and the event can recur, such as for Common and Unscheduled events, the value that is returned is from the latest form instance based on event date.
The variables used are the items in the design, referred to as described in section Addressing Viedoc items.
The operators used are listed in section Operators.
Addressing Viedoc items
An item from a different form (a cross form item) is addressed in Viedoc in the following format: Event.Form.Item. It consists of three identifiers separated by ".", as following:
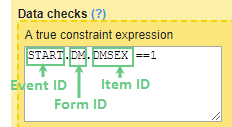
- The event identifier - see Specifying the event below.
- The form identifier - see Specifying the form below.
- The item identifier - see Specifying the item below.
Note!
- An event is any event defined in the study workflow of the study design.
- The JavaScript language definition allows space between keywords, but spaces inside cross form variable are not allowed, because, in order to provide values to execution context, Viedoc parses the whole expression for syntax as specified below.
Specifying the event
The event can be specified in one of the following ways:
EventID
- the Event ID as specified in the Event settings.EventID[StudyEventRepeatKey]
- if the event is recurring and you want to specify a certain occurrence, this is done by writing itsStudyEventRepeatKey
value within [ ].- by using the indexers, for example
EventID$FIRSTn
- to identify the nth initiated event, in case the specified form appears in multiple events. See Relative path section below for a complete description of the indexers.
Specifying the form
The form can be specified in one of the following ways:
FormID
- the form ID as specified in Viedoc Designer under form settings.FormID[FormRepeatKey]
- if the form is a repeating form and you want to specify a particular instance, this is done by writing itsFormRepeatKey
value within [ ].FormID$ActivityID
- optionally, it is possible to specify the activity, in case the respective form appears in multiple activities within the same event.
For exampleDM$MORNING.WEIGHT
- refers to the WEIGHT item in the DM form within the MORNING activity.FormID$ActivityID[FormRepeatKey]
- if you want to specify both the activity and a particular instance of a repeating form, this is specified in this format.
Specifying the item
To access an item in the same form, it is sufficient to use the ItemID
only, there is no need to specify the event and form. In this case, when changing the value of the input item in Viedoc Clinic, the result item (depending on the input item) will update its value at the same time.
In case of using the Event.Form.Item for an item within the same form, when changing the value of the input item in Viedoc Clinic, the result item value will be updated only after saving the form and re-opening it.
To access an item in another form (so called cross form item) it is necessary to specify the event and the form:
EventID.FormID.ItemID
Relative path
It is also possible to access cross form variable using relative path. Some special keywords are used in this case. Keywords that can be appended to StudyEventDefId
. These keywords can also be used without StudyEventDefId
to select any event.
Keyword | Description | Example(s) |
---|---|---|
$FIRSTn |
Selects the first event with the form initiated, n is an optional index and it goes forward (meaning that on a timeline $FIRST2 comes before $FIRST3 ) |
AE$FIRST.AEFORM.AEDATE |
$LASTn |
Selects the last event with the form initiated, n is an optional index and it goes backwards (meaning that on a timeline $LAST2 comes after $LAST3 ) |
AE$LAST.AEFORM.AEDATE |
$PREVn |
Selects the previous event with the form initiated, n is an optional index and it goes backwards (meaning that on a timeline $PREV2 comes after $PREV3 |
WEIGHT < $PREV.DM.WEIGHT + 10 |
$THIS |
Selects the current context event |
Example of using the optional indexer
Here comes an example of using the optional indexer (n in the above table).
On the Add patient form, we have a text item that should have the latest non-blank value of another text item (called NAME) that is present on a form (called PROFILE) which is present on all scheduled (the first one called START) and unscheduled events.
The function below can be used on the item of the Add patient form:
if($LAST.PROFILE.NAME != null) return $LAST.PROFILE.NAME;
if($LAST2.PROFILE.NAME != null) return $LAST2.PROFILE.NAME;
if($LAST3.PROFILE.NAME != null) return $LAST3.PROFILE.NAME;
if($LAST4.PROFILE.NAME != null) return $LAST4.PROFILE.NAME;
if($LAST5.PROFILE.NAME != null) return $LAST5.PROFILE.NAME;
if(START.PROFILE.NAME != null) return START.PROFILE.NAME;
return 'NOT SET';
This will allow for the item being saved blank for the last 4 events, or else fallback to the value of the item on the start event.
Cross event date
Accessing the date of another event uses same principle as any cross form variable, uses a fixed form id $EVENT
and item id EventDate
.
For example, AESTDT > BL.$EVENT.EventDate, means that the start date of AE must be after BL event date.
Operators
The below JavaScript operators are used:
- Arithmetic Operators:
- + (addition)
- - (subtraction)
- * (multiplication)
- / (division)
- Comparison:
- == (equal to)
- != (not equal to)
- < (less than)
- > (greater than)
- <= (less than or equal)
- >= (greater than or equal)
- Boolean logic:
- && (AND)
- || (OR)
- ! (NOT)
Data types
The following table lists the Viedoc items together with their JavaScript data types and default values.
Viedoc item | JavaScript data type | Default value |
---|---|---|
Single line text | String | null |
Number | Number | null |
Date |
Date object. Note! JavaScript counts months from 0 to 11. Thus, January is 0 and December is 11. Note! In an expression such as Note! Due to this, the date field will not behave as a date object when used in JS expressions. To account for this, the date string has to be converted to a date object by adding it as input for new Date().
|
null |
Time | Date object | null |
Paragraph text | String | null |
Checkbox | Array of string/number* | [ ] |
Radio button | String/Number* | null |
Dropdown | String/Number* | null |
File |
Object with following members:
|
null |
Range | String representation of a range object (see more details in section Range item specific functions and properties). | null |
*The item type for checkbox, radio button or dropdown menu is usually number, unless any of the choice codes is not a number.
Pass by value vs. pass by reference
In JavaScript data is passed in two ways: by value and by reference, respectively.
Passed by value
The following JavaScript data types are the ones that are "passed by value":
- boolean
- null
- undefined
- string
- number
This means that, when comparing two variables of the above mentioned types, their values will be compared. It means that, for example if we have the following code:
var a = 3;
var b = 'def';
var x = a;
var y = b;
...then a
will get the value 3, b will get the value 'def', x
will get the value 3, y
will get the value 'def'. Variables a
and x
have the same value (3), and b
and y
have the same value ('def'). Still, all the four variables are independent. If we continue now and change the value of a
to 5, this will have no impact on var x
, which still has the value of 3.
Pass by reference
The following JavaScript data types are "passed by reference":
- array
- function
- object
This means that variables of these types get a reference to a certain value, not the value itself.
See Data types section for information on which Viedoc items are objects.
The date is always an object in JavaScript. If we have for example, the following:
var d=new Date();
var c=d;
d.setDate(10);
...then variable d
is created as a date object, variable c
is assigned to reference to the same value as d
, and then each and every time we change d
, c
will dynamically change as well, as it references the same value as d
does. So, when we set d
to the 10th of the current month, c
will automatically get the same value.
System variables
When an expression is evaluated in a form context, following variables are accessible:
Variable name | Data type | Default value |
---|---|---|
ItemId , as configured in Viedoc Designer. |
As specified in Data types table, depending on the item type. | As specified in Data types table. |
SubjectKey |
String | null only for add patient. |
SiteSubjectSeqNo |
Number | Sequence number of the subject in the site (starts with 1). |
StudySubjectSeqNo |
Number | Sequence number of the subject in the study (starts with 1). |
SiteCode |
String | The site code as set in Viedoc Admin. |
CountryCode |
String | Two letter International Organization for Standardization (ISO) country code of the site. |
StudyEventDefId |
String | The ID of the study event as specified in the study workflow in Viedoc Designer. |
StudyEventType |
String | "Scheduled", "Unscheduled" or "Common" |
StudyEventRepeatKey |
String | The number that identifies a specific recurrence of a study event that is repeating (such as recurring events or common events). |
FormDefId |
String | The ID of the form as specified in Viedoc Designer > forms > settings. |
FormRepeatKey |
String | Counter that identifies the specific instance of a repeating form within a specific activity. |
SubjectFormSeqNo |
String | Counter that uniquely identifies the instance of a specific form on a subject level, that is, it starts with 1 and it is incremented each time a new instance of the form is created for that subject. See Form sequence numbers below for more details. |
OriginSubjectFormSeqNo |
String | For a copied form instance, it identifies the form instance from which data was copied for the first time. For the first instance of the form (not copied) it gets the value of the SubjectFormSeqNo . See Form sequence numbers below for more details. |
SourceSubjectFormSeqNo |
String | For a copied form instance, a counter that identifies the source of a copied form instance (the form instance the data was copied from). It gets the value of the SubjectFormSeqNo from which the form instance was copied. For the first instance of the form (not copied) it is empty (null). See Form sequence numbers below for more details. |
EventDate |
Date object | Current date for common events, all other current events date. |
ActivityDefId |
String | The ID of the activity as specified in the study workflow in Viedoc Designer. |
Note! Please note that a double underscore must be used. |
Date object |
Date types: 0. Date only for example Numeric with decimals |
BookletStatus |
String |
For PMS studies only! The status of the booklet, can be one of the following:
Default status is NotSubmitted. This system variable can be accessed by 3 part expression <event identifier>.$EVENT.BookletStatus, for example $THIS.$EVENT.BookletStatus, V1.$EVENT.BookletStatus, or $PREV.$EVENT.BookletStatus. |
Statistics variables
Below is a table of statistics variables that can be used in study designs.
For the variables, all values are current values at the time of evaluation expression. This means that the value (the total number, for example) is tied to the specific event.
Variables are available in the following format: <Event Identifier>.$STATS.<variable as mentioned in the table below>
For example: $THIS.$STATS.QueryRaisedCount
Variable name |
Data type | Description |
---|---|---|
|
Number | The total number of queries raised in the event. |
|
Number | The total number of queries resolved in the event. |
|
Number | The total number of queries rejected in the event. |
|
Number | The total number of queries approved in the event. |
|
Number | The total number of queries closed in the event. |
IncompleteFormsCount |
Number | The total number of incomplete forms in the event. |
|
Number | The total number of unlocked forms in the event. |
|
Number | The total number of forms where the CRA is not checked in the event. |
|
Number | The total number of forms not reviewed by the data manager in the event. |
|
Number | The total number of forms that have not been SDV'd in the event. (Where SDV is Source Data Verification.) |
|
Number | The total number of forms that have not been signed by the investigator in the event. |
Note! These statistics variables are not supported for any summary format. Expressions containing these variables are not evaluated when the counts are updated or changed.
Form sequence numbers
The following form sequence numbers are used to make it easier to track different form instances at subject level, which are useful especially for the form instances initiated by copying the data from previous event.
- FormRepeatKey - Counter that identifies the specific instance of a repeating form within a specific activity. This is available in the export output for Viedoc output version 4.39 and onwards.
- SubjectFormSeqNo – Counter that uniquely identifies the instance of a specific form on a subject level, that is, it starts with 1 and it is incremented each time a new instance of the form is created for that subject. This is available in the export output for Viedoc output version 4.51 and onwards.
- OriginSubjectFormSeqNo – For a copied form instance, it identifies the form instance from which data was copied for the first time. For the first instance of the form (that is, not copied) it gets the value of the
SubjectFormSeqNo
. This is available in the export output for Viedoc output version 4.51 and onwards. - SourceSubjectFormSeqNo – For a copied form instance, a counter that identifies the source of a copied form instance (the form instance the data was copied from). It gets the value of the
SubjectFormSeqNo
from which the form instance was copied. For the first instance of the form (that is, not copied) it is empty, that is, null. This is available in the export output for Viedoc output version 4.51 and onwards.
The example below illustrates how the values for these sequence numbers are assigned. The demo form used is set as repeatable and copyable and is included in Visit 1, Visit 2 and Visit 3.
We perform the following actions in Viedoc Clinic:
These sequence numbers are available to be used within expressions only to get the value of the sequence number for a specific form instance, that is, by using {SubjectFormSeqNo}, {OriginFormSeqNo}, {SourceFormSeqNo}.
In the above example, the form Summary format was configured by using these sequence numbers as below:
Form Repeat Key {FormRepeatKey}, SubjectFormSeqNo {SubjectFormSeqNo}, OriginFormSeqNo {OriginFormSeqNo}, SourceFormSeqNo {SourceFormSeqNo}
Notes!
- Only the FormRepeatKey is used to identify a specific instance of the form in data mapping for data import,as well as in the item identifier used in JavaScript (for example EventID.FormID$ActivityID[FormRepeatKey].ItemID).
- When resetting a form, the sequence numbers are still allocated to it, and the next available ones are used for the new instances.
In the excel export output, these form sequence numbers allows to track, for the form instances that were initiated by copying data from previous events, where the data originates from, as below:
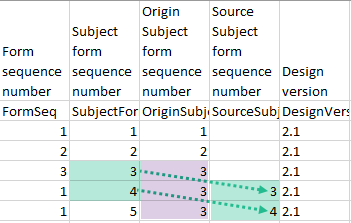
Analyzing the values of the form sequence numbers, only the form instances that were initiated by copying the data from previous visits have values populated in the Source Subject form sequence number column, that is, the last two rows in the example. The data was copied from the form instance having the same Subject form sequence number value, highlighted in green in the above image. The form instance that the data was copied for the first time is identified by the value of the Origin Subject form sequence number, that is, "3" in our example.
Expressions
An expression in Viedoc is a JavaScript function body written in ECMAScript 5.1 standards.
For example:
return 2;
var a=2;
var b=3;
return a+b;
Expressions can be written as single statement. Viedoc converts them into a function body.
expression
is converted to return (expression)
, for example:
2
converts to
return (2);
or
VSDT <= now()
converts to
return ( VSDT <= now());
Important! When using loops (for, for/in, while, do/while
) consider the following:
- Do not copy code (from internet) without reviewing it first and checking what it does.
- Make sure that you don't have endless loops.
- Consider browser compatibility when using JavaScript functions.
- Avoid inline/recursive functions, as they could cause memory problems in the system, both on the client side as well as on the server side.
JavaScript expression editor
The JavaScript expression editor is an additional help tool when writing JavaScript expressions. These are the main features of the editor:
- Autocomplete and lookup
- Error highlighting
- Links to eLearning sections where you can read more about Viedoc-provided items and functions
The JavaScript expression editor is available in all locations in Viedoc where it is possible to enter an advanced condition as a JavaScript expression, for example in form settings, alerts settings, edit check expressions, and subject status settings.
To open the editor, click the JS button in the JavaScript text editor field:
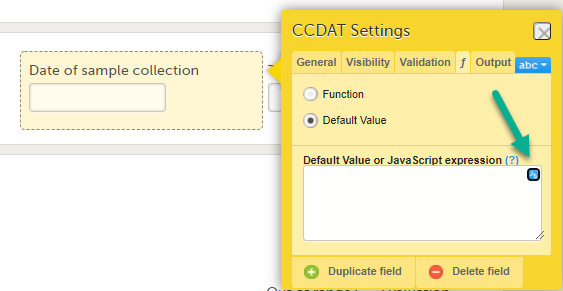
The editor opens:
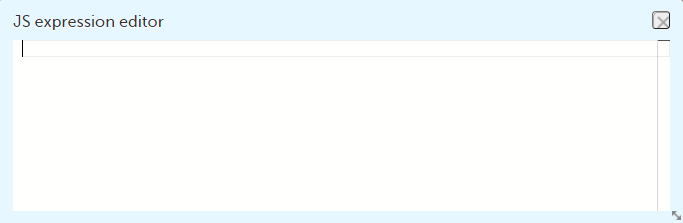
You can move the editor window around on your screen, and you can resize it by using the double-pointed arrow in the lower right corner.
Autocomplete and lookup
The JavaScript expression editor supports autocomplete and lookup for:
- Accessing cross-form variables
- Accessing context form variables
- Viedoc-provided helper functions, for example
age
,date
, andtoday
When you start typing in the JS expression editor window, a help pane displays autocomplete suggestions. To read more about an item in the list of suggestions, hover over it with the mouse and then click on the >
symbol in the top right corner of the pane or type Ctrl+space.
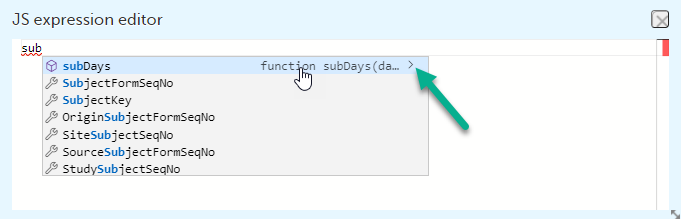
The help pane then displays information about the syntax of the item and parameters, return values etc.:
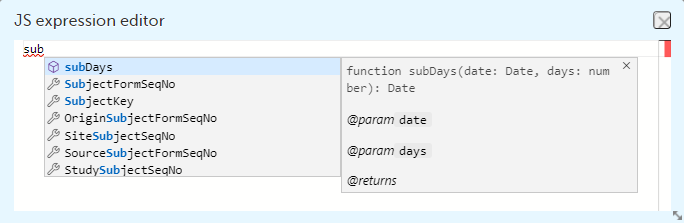
For items that are radio buttons, checkboxes, or dropdown menus, the help pane displays information about the code list values:
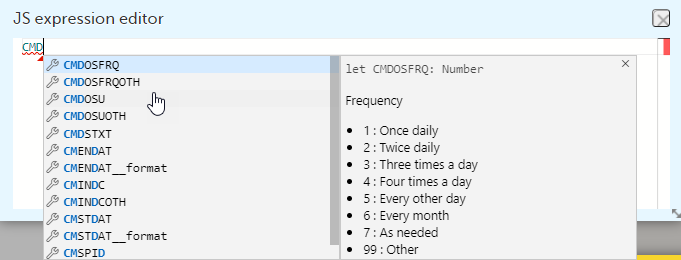
Tip! To see a list of all available items, type Ctrl+space or start typing.
Error highlighting
If there is an error in the code, it is underlined in red. To find out more about the error, hover over it with the mouse.
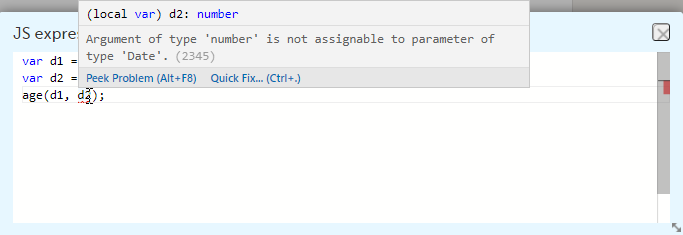
Click on Peek Problem or type Alt+F8 to display the error information inline in the editor window. To receive suggestions on how to fix the error, click Quick Fix.
Note! In some cases, the JavaScript expression editor incorrectly flags expressions as faulty. However, the validation that is performed when you save your changes will correctly validate your expression. For more information, see Unsupported expression types.
Links to eLearning
For the Viedoc-provided items and functions, the help pane can contain a link to the section of the eLearning that has more information about the specific item or function.
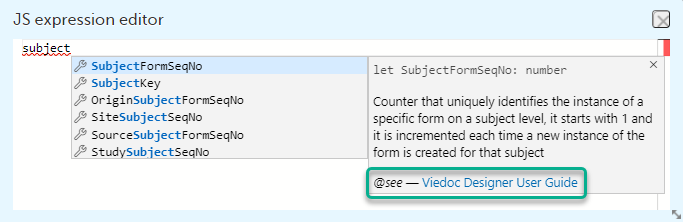
Unsupported expression types
The following types of expressions are not supported by the JavaScript expression editor. However, they are still valid, and your study design will be correctly validated when you save the changes.
- Referencing cross-form variables using activity names, for example
[$ActivityDefId]
- Referencing cross-form variables using indexers, for example
$FIRST[index]
Boolean expressions
The boolean expressions are the expressions that return a boolean value, either true
or false
. They are used in the Visibility and Validation.
true
- everything with a real value istrue
, such as:100
"Hello"
"false"
7 + 1 + 3.14
5 < 6
false
- everything without a real value isfalse
, such as:0
Undefined
null
""
For example, a validation expression to check that the weight is > 65 for males ('M') and > 45 for females. Gender (ItemID=GENDER
) is collected in Patient Information form (FormID = PI
) withing the Screening event (EventID = SCR
) and weight (ItemID = WEIGHT
) is collected in Demographics form in each event. Below is the edit check written for the WEIGHT
item:
if (SCR.PI.GENDER == 'M')
return WEIGHT > 65;
else
return WEIGHT > 45;
Date comparison
Dates in JavaScript are objects and the available comparators are:
- >
- >=
- <
- <=
If you need to use "==" or "!=", convert to string and compare, as in the example in the image below, except for the case when checking for NULL. When checking for NULL, do not convert as this would lead to a change of the value during conversion from NULL to something different.

Note! Remember to check for NULL before invoking any function on an object.
See the Pass by value vs. pass by reference section for an explanation on working with objects.
Checking if two dates are the same
For checking if two dates are the same in Viedoc, the function below can be used:
function sameDay(d1, d2) {
return d1.getFullYear() === d2.getFullYear() &&
d1.getMonth() === d2.getMonth() &&
d1.getDate() === d2.getDate();
}
return sameDay(Date1, Date2);
where Date1 and Date2 are the Item IDs used in Viedoc for the date items being compared.
Finding the earliest/latest date
For getting the latest date out of, for example, three different dates, the following can be used:
if(DATE1 != null && DATE2 != null && DATE3 != null)
{
var latestDate = Math.max(DATE1.valueOf(), DATE2.valueOf(), DATE3.valueOf());
return new Date(latestDate);
}
return null;
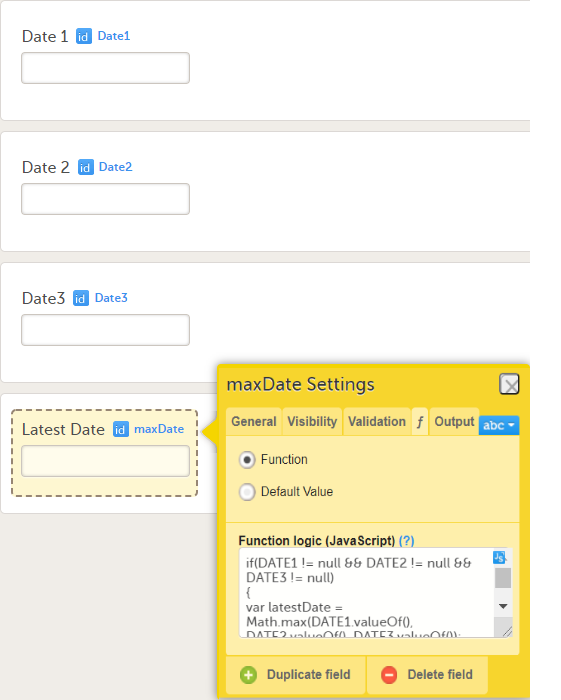
In a similar way, for getting the earliest date, the Math.max
function should be replaced with Math.min
:
if(DATE1 != null && DATE2 != null && DATE3 != null)
{
var earliestDate = Math.min(DATE1.valueOf(), DATE2.valueOf(), DATE3.valueOf());
return new Date(earliestDate );
}
return null;
Note! The .valueOf() function does not work for primitive data types. The javascript editor supports the function, but it should not be used for primitive data types.
File properties
The metadata values of the file datatype can be accessed in expressions, as shown in the image:

Default value
The default value expressions are executed and the resulting value is set only when the form is initialized.
Note! If there is visibility condition set on item with function or default value, whenever item becomes hidden, their value is reset to default value.
Viedoc provided functions
Function | Description | Implementation |
---|---|---|
date(dateString) |
Converts date string to JavaScript date object. The dateString must be in "yyyy-mm-dd" format. | |
today() |
Returns current site date. | |
now() |
Returns current site date and time (in site timezone). | |
addDays (date, days) |
Add the specified number of days to the specified date object. | |
bmi ( weightInKg, heightInCM ) |
Returns the bmi (body mass index) calculated based on the provided weight (in kg) and height (in cm). | function bmi(weight, height) { |
days ( DateA, DateB ) |
Calculates the number of days between 2 dates provided as input parameters: Note! The result is always rounded to the nearest integer (see function implementation below), and when using at least one input parameter that contains both date and time, this means that, for example, for a difference of 1.3 days the function will return 1, and for a difference of 1.7 days the function will return 2. |
function days(endDate, startDate) { |
hours( DateTimeA, DateTimeB ) |
Calculates the number of hours between 2 "date and time" items provided as input parameters: DateTimeA and DateTimeB , as DateTimeA - DateTimeB. |
function hours(endDateTime, startDateTime) { |
minutes( DateTimeA, DateTimeB ) |
Calculates the number of minutes between 2 "date and time" items provided as input parameters: DateTimeA and DateTimeB , as DateTimeA - DateTimeB. |
function minutes(endDateTime, startDateTime) { |
Array.contains function
When working with check box items (or array types in general), a special JavaScript function is used to evaluate visibility conditions and edit checks: [].contains( x )
This function checks if an array type contains a value and evaluates as either true or false.
Examples:
- If you want to test if a check box item has one of its options selected, you can write the following:
ItemID.contains(CodeListValue)
Where ItemID is the ID of the check box and CodeListValue is the ID of one of the code list options for the check box. If code list is selected, then the function evaluates as true.
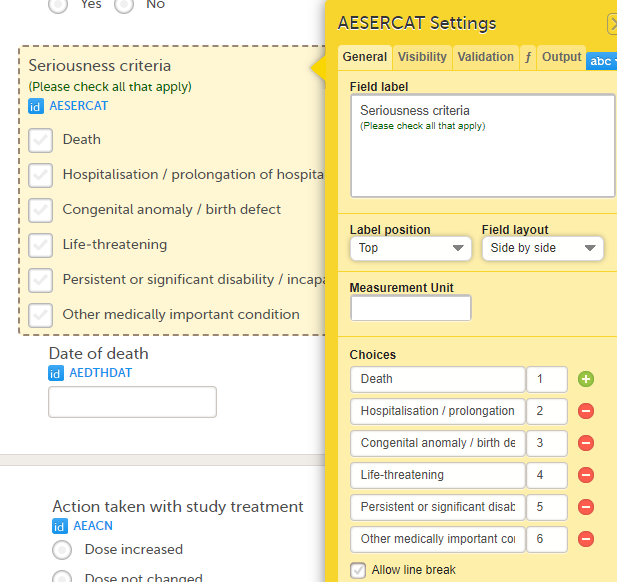
- You may have a "None of the above" option, where it doesn't make sense to allow multiple selections. In that case, you can add the following check:
if(ItemID.contains(3))
return !ItemID.contains(1) && !ItemID.contains(2);
else return true;
In the example above, 3 is the code list for the none of the above options and 1 and 2 are the code lists for the other options in the list. Also note the use of the exclamation mark, which means "NOT" as in, the array should not contain this code list value.
- You may have something you would like to only show for a specific event or activity. You can define a list of the events or activities as an array, and then use that array for the evaluation:
var skipActivities = ['V1A1', 'V2A1', 'V3A1'];
skipActivities.contains(ActivityDefId);
If the above were used as an advanced visibility condition for a group, then the group would only show if it existed within that Activity.
Range item specific functions and properties
The range item is the string representation of a range object. The functions that can be used to convert the respective string to a range object and vice versa are described below.
The properties available for the range object are:
Property | Description | Type |
---|---|---|
RangeObject*.Lower |
the lower limit of the range | number |
RangeObject*.LowerFormat |
the number of decimals used for the lower limit of the range | number |
RangeObject*.Upper |
the upper limit of the range | number |
RangeObject*.UpperFormat |
the number of decimals used for the upper limit of the range | number |
RangeObject*.Comparator |
the comparator used to define the range. The available comparators are:
Note! When using the comparator in functions, make sure to write it between quotes, for example |
string |
RangeObject*.StringValue |
the string representation of the respective range item | string |
*RangeObject can be obtained as output of the first two functions described below.
The functions available to be used in conjunction with the range item are described in the table below.
Function | Input | Output | Example |
---|---|---|---|
parseRangeValue(value) |
value as string | range object, null if the input is empty or if it cannot be parsed |
|
createRangeValue(lower, comparator,upper) |
- lower limit as string or float - comparator as string - upper limit as string or float |
range object, null if the input is empty or if it cannot be parsed | createRangeValue("1.3", "InclusiveInBetween", "2.0") |
getRangeValue(rangeValue) |
rangeValue as range object | string representation of the range defined by the input range object |
|
inRange(rangeValue, numericValue) |
- range value as string or range object - numeric value |
- boolean value showing if the input numeric value is within the input range (true) or not (false):
|
|
This example code populates a range item:
var rangeValue = createRangeValue("1.3", "InclusiveInBetween", "2.0");
return getRangeValue(rangeValue);
Math library
The ECMAScript contains math objects that can be used for mathematical calculations.
Function in item settings
For a Viedoc item it is possible to write a function in order to assign a particular value to it, either by writing a function that would return a result depending on other items/conditions, or by assigning a default value, as illustrated in the image:
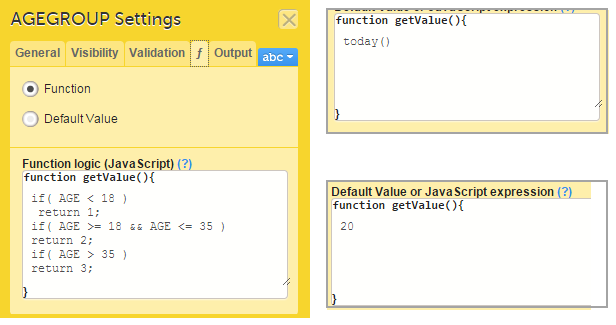
Note!
- Functions that returns a value to form item must match the data type as specified in Data types section.
- Despite what was earlier mentioned about the matching of data types, nothing will prevent you from writing a non-matching function. Please note that, in case of returning a number with decimals or a date object to a text field, the decimal separator or the date format will be in the format configured on the server, for those cases when the respective function is executed on server side, that is:
- when applying a new revision.
- for the "auto-update" forms.
- Functions are not supported in Viedoc Me forms.
Debugging your expression
To debug you can use the following in your expression:
debugger;
statement.
The above statement will have effect only when opening the developer tools of the browser while entering data to the respective form in Viedoc Clinic.
Note!
- When debugging, you cannot use single line statements.
- You cannot debug the event/activity visibility expression as they are run on server.
Validation
During Save changes and VALIDATE operations in Viedoc Designer, the expressions are validated using a compiler, which would find most of the errors. However, since JavaScript is a dynamic language, not everything can be validated.
For example, AGE.foo ()
will not throw an error, because AGE
is a variable in a form and the compiler does not know its type.
Important! The designer must test the expression in all the possible paths using either preview or Viedoc Clinic. |
Examples/Use cases
See the following lessons for some examples of using JavaScript:
- Calculating the age of a subject
- Adding an auto counter in common events
- Using advanced visibility conditions
- Calculating the difference between two time variables
- Controlling the format of a date/time variable
- Configuring a check to apply to a specific event or activity
- Checking if date only is entered for a Date and Time variable
- Controlling the number of decimal digits in a number item
- Extracting descriptions from the form link item