Exporting data via Viedoc's web API
This lesson explains how to export data via Viedoc's web API. You will be shown three examples: Windows command prompt, Python, and R.
Note! You must have the API Manager role in order to see the API configuration field.
Configuring the API client
Important! To export data, enable the Export scope and to select the correct Status while configuring the API client. We have two modes: demo and production.
Demo – Used to access sites that operate in Demo/Training mode
Production – Used to access sites that operate in Production mode
After creating the API client, take note of the following information, as it is needed in subsequent steps:
Client secret – Needed to obtain the token. Tip! Make sure you copy it, because it is shown only once. If needed, you can regenerate it.
Client ID – Needed to obtain the token.
Token URL – Used for obtaining the token, which is needed to authorize all subsequent API calls.
API URL – All other API calls are made to this base URL with varying endpoints.
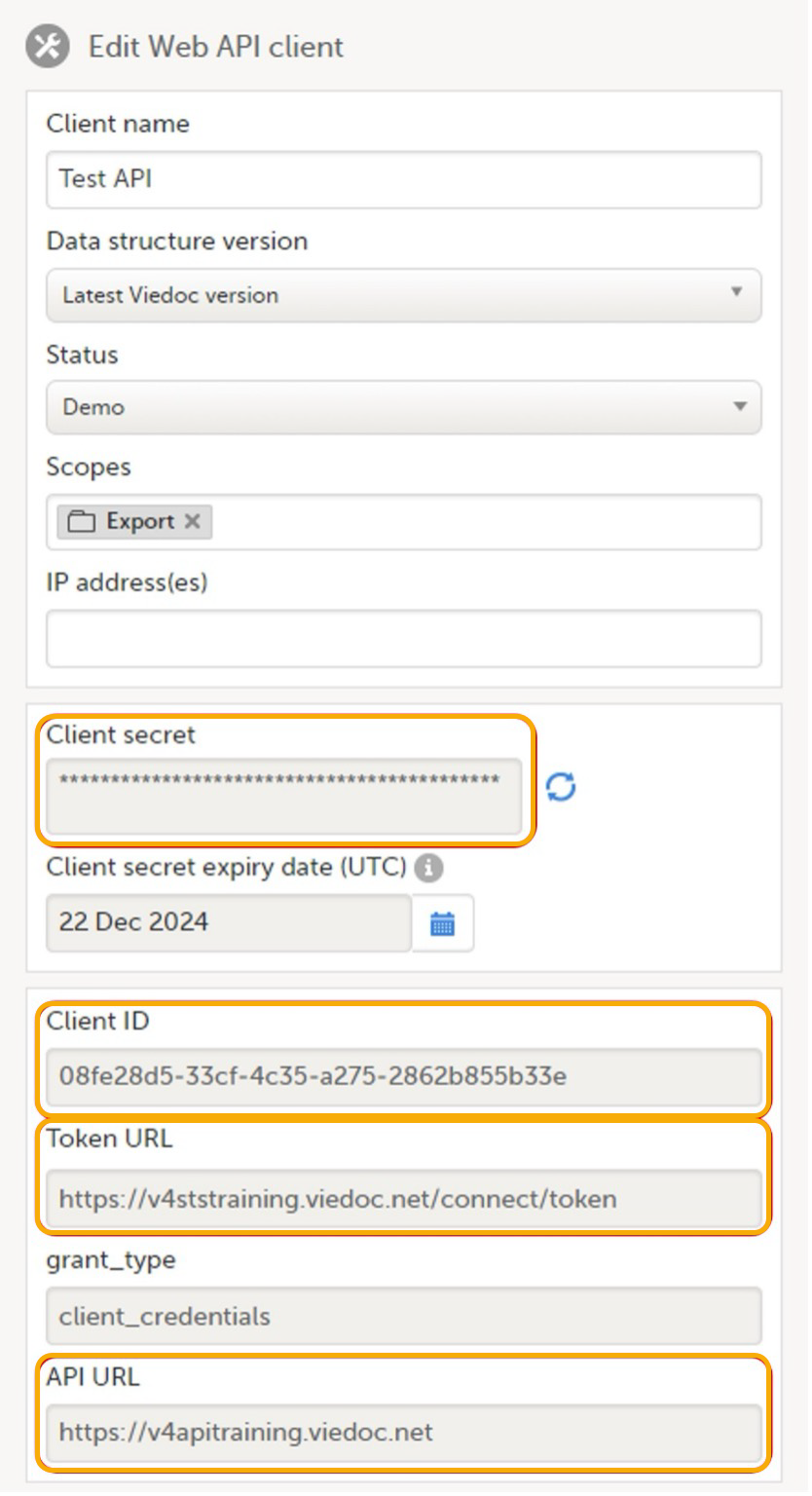
For more information on how to configure the API client, select this link: API configuration.
Examples
Windows command prompt
This section describes the steps to export the data using the Windows command prompt.
1 |
Obtain the token Note! Replace "xxxx" with your Client ID and "yyyy" with your Client Secret. The TokenURL is obtained from Viedoc Admin. This is an example of template including the output: ![]() |
|
2 |
Start the export process Note! Replace This is an example of the template including the output:
|
|
3 |
Check the export process To check the export process, you can use the following code as a template:
Note! Replace This is an example of the output:
|
|
4 |
Download the export To download the export, you can use the following code as a template:
xxxx with the token and the APIURL with the API URL obtained in Viedoc Admin. Replace yyyy with the export ID. Finally, the path where the file will be saved needs to be specified along with the name and file extension (.zip for CSV exports, .xlsx for Excel, and .xml for XML).
This is an example of the template including the output: ![]() |
Python
This section will take you through the steps to export data using Python.
Note! This example uses the requests package for Python. Ensure that you have it installed before running the code below. To install the requests package open command prompt or terminal and type pip install request.
1 |
Obtain the token
This is an example of how to structure the request for a token in Python: ![]() |
|
2 |
Start the export To start the export process, you can use the following code as a template:
Note! The This is an example of the start of the export process: ![]() |
|
3 |
Check the export status To check the export status, you can use the following code as a template:
Note! The above code checks for the completion of the export process every 4 seconds. ![]() |
|
4 |
Download the export To download the export, you can use the following code as a template:
Note! You need to specify the file path where you will save the file, as well as the file name. ![]() |
R
This section will take you through how to export data using R.
Note! This example uses the httr and jsonlite packages for R. You need to install them before running the code in this example. To do so, type (install.packages(c("httr", "jsonlite"))
into your R console. You only need to do this once.
1 |
Obtain the token To obtain the token, you can use the following code as a template:
Note! Replace the This is an example of how to structure a token request: ![]() |
|
2 |
Start the export process To start the export process, you can use the following code as a template:
Note! The ![]() |
|
3 |
Check the export status To check the export status, you can use the following code as a template:
Below is a screenshot of the export status: Note! The above code checks for the completion of the export process every 4 seconds. |
|
4 |
Download the export To download the data export, you can use the following code as a template:
Note! You need to specify the file path where you will save the file, as well as the file name. Below is a screenshot of the export download: ![]() |
|
5 |
Analysis
This is a screenshot of the exported data for analysis: ![]() |