Viedoc Web API
Introduction
The Viedoc Security Token Service (STS) is a centralized service for issuing and validating access tokens for use with APIs in the Viedoc eClinical suite. In other words, the Viedoc STS is an Identity Provider (IdP) that provides authentication services for so-called principals (or security principals). Principals can be computers, services, computational entities such as processes and threads, or any group of such things.
The Viedoc STS is separate from the Viedoc REST API.
Note! Viedoc STS is not used for the Viedoc WCF API.
The Viedoc STS consists of a web service with endpoints for getting and validating tokens. The Viedoc STS also exposes metadata (as JSON documents). This metadata is used by clients and APIs for self-configuration when communicating with the service.
The Viedoc STS follows the OAuth 2.0 standard, which is the industry standard for authentication and authorization for web applications and mobile applications. For more information, see The OAuth 2.0 Authorization Framework.
The Viedoc STS is publicly accessible.
Authorization in Viedoc REST API
The Viedoc REST API requires authentication and authorization on every request. To achieve this, an access token must be included in the request as an HTTP authorization header. The token should be supplied with the Bearer authentication HTTP scheme.
The Viedoc REST API only accepts tokens issued by the Viedoc STS.
Receiving tokens from Viedoc STS
Grant types
Viedoc STS uses a non-interactive grant type called Client Credentials. This grant type is easy to use and is specifically made for scenarios where there is no user ID. In other words, this grant type lets you retrieve tokens for machine-to-machine communication only.
Credentials
In a token issue request to the Viedoc STS, you need to supply a set of credentials, that is, keys and values, as form data in an HTTP POST request. These are examples of such credentials:
Key | Value | Description |
---|---|---|
client_id |
viedoc-web |
The client ID to issue a token for. |
client_secret |
viedoc-secret |
The client secret (=password) for the given client ID. |
grant_type |
client_credentials |
The type of authentication. |
Note! For information about the Web API client (how to obtain the client id and client secret), see API Configuration.
Approach 1: Plain HTTP client
To retrieve an access token, make a POST request to the "token endpoint" of the Viedoc STS, located at http://<base-url>/connect/token
, with the keys and values (described in the section Credentials) above the POST request body.
Approach 2: Identity Model library
When using the Identity Model in .NET Framework or .NET Core, you can use extension methods on HttpClient
. The following example shows a suitable method for working with the Client Credentials grant type:
|
Running the code above will generate a strongly typed response containing either an error or, if successful, an access token to use in requests to the Viedoc API.
For more information, see the documentation of the .NET integration library IdentityModel
.
Validating tokens
Tokens must be validated on several parameters for your application to trust that the tokens have not been tampered with. The main validation criteria are:
- the audience claim
- the scope claims
- the issuer claim
- the cryptographic signature
You can validate tokens with one of these two methods:
- Offline - by the receiving application/API. This method is strongly preferred for performance reasons.
- Online - by sending the token back to the STS for verification, so called introspection. This method should only be used when no other options are available.
The validation can be done automatically with the use of convenience libraries. See examples for .NET and JavaScript below.
Offline validation with IdentityModel in .NET
When building a Web API based on ASP.NET Core, token validation can be done via extensions to the request pipeline. The request pipeline automatically works with the built-in authorization system, that is "principals", in ASP.NET Core. When you set up the pipeline in your Startup.cs
file, you can add JWT Bearer authorization as in the following example.
The first step in the example adds the NuGet package Microsoft.AspNetCore.Authentication.JwtBearer
.
|
For more information, see Overview of ASP.NET Core Authentication | Microsoft Docs.
Offline validation with JavaScript
You can use the oidc-client
JavaScript library to:
- download metadata from the STS
- validate tokens
- extract claims
The library is available as an NPM package, and it is primarily intended for use in JavaScript clients. For more information, see the oidc-client
documentation.
Web validation
The web site https://jwt.io offers the possibility to validate tokens. Simply paste your token into the field Encoded and then the field Decoded will display information about your token.
Online validation (introspection) with the STS
Sending the token back to the STS for validation should be seen as a last resort, in cases where, for some reason, it is impossible or infeasible to validate the token with an offline method.
For more information, see Introspection Endpoint.
Viedoc Web API documentation
The Viedoc Web API is documented on the Viedoc API swagger page. The Viedoc.API swagger page is accessible at:
<API URL>/swagger, where the API URL depends on the environment. For example, see the following link:
https://v4apitraining.viedoc.net/swagger/
For more information, see the instructions below on how to access the API URL for your environment.
To view the Viedoc Web API swagger page, you need the API URL in Viedoc Admin.
Note! You must have the API Manager user role to see the API configuration field.
To view the API URL in Viedoc Admin:
1 | On the Viedoc landing page, select the Admin icon to open Viedoc Admin. |
2 |
Open the study that you would like to work with and select the Edit button in the API configuration field to open the API configuration dialog. ![]() |
3 |
On the Web API client tab, select Edit for the API Client you want to access. ![]() |
4 |
The Edit Web API client dialog opens: ![]() Using the example above, add /swagger to the API URL: https://v4apitraining.viedoc.net/swagger to open the swagger page: ![]() |
Viedoc Web API version
The Viedoc Web API swagger page contains information about how to connect to and interact with Viedoc using the Viedoc Web API, including methods, parameters and response messages.
Updates to the Viedoc Web API considered as breaking changes will always be introduced in a new API version. To ensure backward compatibility is maintained, you need to specify the API version to be used.
Important! If the API version is not specified either in the api-version query or in the Accept-Version header, the latest available version for the endpoint will be used. Different versions will also be available for different endpoints. |
In the api-version
query field or in the Accept-Version
header field you can specify which API version should be used to connect to and interact with Viedoc.
To specify the API version, enter the date of the version required into the api-version
query field or into the Accept-Version
header field as shown in the example below for the dataexport/start endpoint.
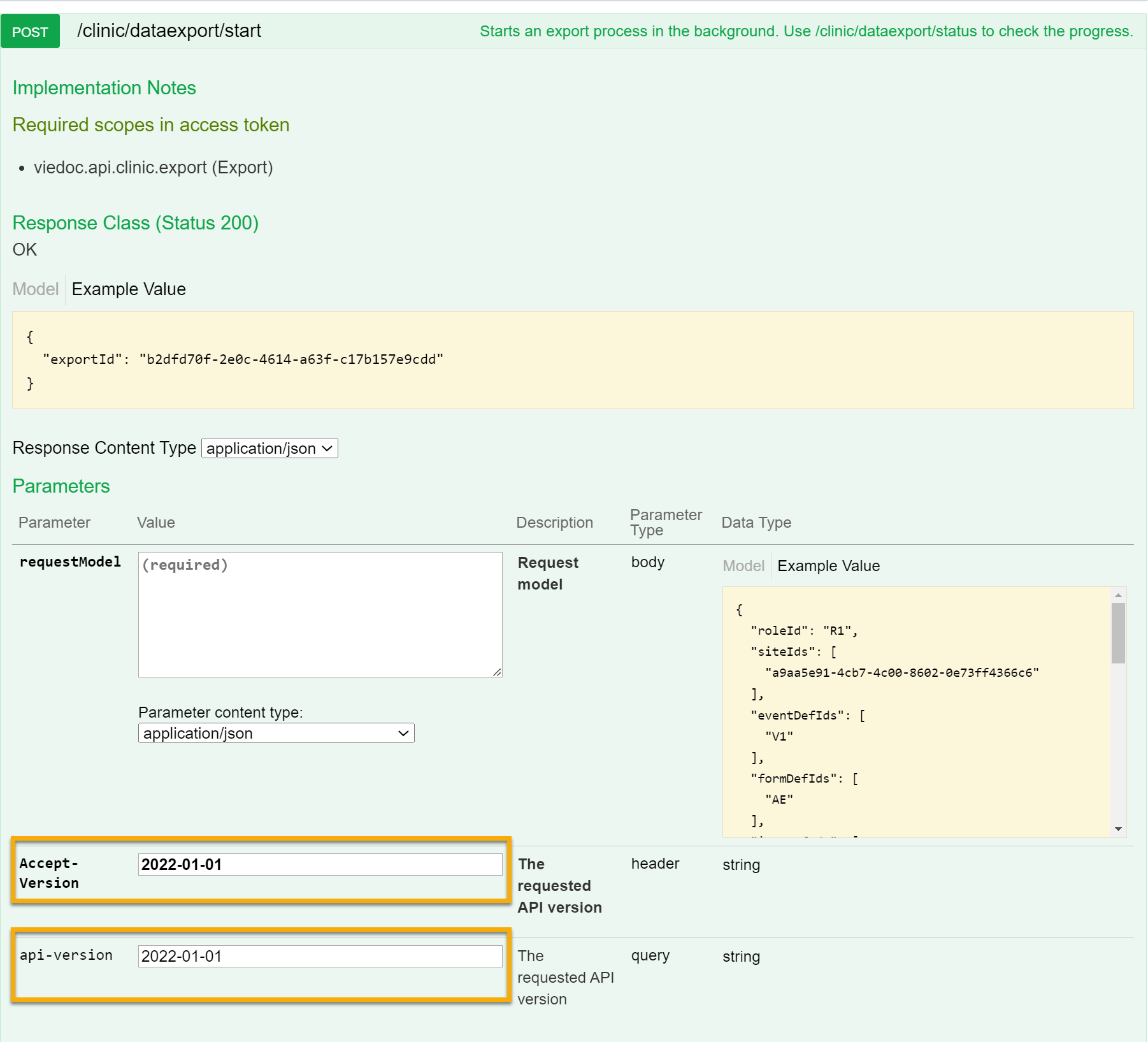
Available versions |
|
Viedoc Web API version Changes from previous version: N/A- first version. |
2022-01-01 |
Further reading
- The
IdentityServer
documentation - The
IdentityModel
documentation