Viedoc WCF API
Introduction
This document contains information on connecting your development environment or any other system to the Viedoc public web service using the Windows Communication Foundation (WCF) standards.
The Viedoc public Application Programming Interface (API) is a Simple Object Access Protocol (SOAP) over a Hypertext Transfer Protocol (HTTP) service. The API can be reached at: https://[VIEDOC_HOST]/HelipadService.svc
A wsdl metadata file can be downloaded from: https://[VIEDOC_HOST]/HelipadService.svc?wsdl
For the EU:
https://v4api.viedoc.net/HelipadService.svc?wsdl
https://v4apitraining.viedoc.net/HelipadService.svc?wsdl
For Japan:
https://v4apijp.viedoc.net/HelipadService.svc?wsdl
https://v4apitrainingjp.viedoc.net/HelipadService.svc?wsdl
https://v4apistagejp.viedoc.net/HelipadService.svc?wsdl
For China:
https://api.viedoc.cn/HelipadService.svc?wsdl
https://apitraining.viedoc.cn/HelipadService.svc?wsdl
For the USA:
https://api.us.viedoc.com/HelipadService.svc?wsdl
https://apitraining.us.viedoc.com/HelipadService.svc?wsdl
Contact Viedoc Technologies for information about which host to connect to.
See Guide to Viedoc server instances for more information.
Methods
Token
Description
The Token
method is used for authenticating the client. This method must be called to receive a token for authenticating all subsequent requests.
To authenticate the client, the following must be provided:
-
An active Client ID, a client ID (GUID) linked to a specific study in Viedoc. The client ID is linked to either the demo or the production study.
-
A Viedoc user name and password. To submit data into Viedoc, you need access to the study in Viedoc and to the study site with a role that allows data entry.
Note! You can only access the API configuration window and create an API client ID if you are assigned the role API Manager. All the pending role invitations for a user are automatically approved when the Token
/GetToken
method is used.
For information about how to obtain a client ID, see API configuration.
C# Syntax
ApiTokenModel tokenModel = Token(ApiAuthenticationModel loginModel);
Parameters
The Token
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
loginModel |
ApiAuthenticationModel |
A collection of authentication information. See section 3.1 ApiAuthenticationModel for a description. |
Returns
The Token
method returns an ApiTokenModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Token |
string |
A new authentication token that can be used for authentication in subsequent requests |
Result |
ApiResultType |
Defines the type and status of the result returned from method invocation. See section 3.2 ApiResultType for details |
ErrorCode |
int |
In case of an error result: contains an integer specifying the type of error. See section 4 Error codes for details. |
ErrorMessage |
string |
In case of an error result: human readable description of the error |
ExpiryDateTime |
DateTime |
Token expiration date and time |
Example HTTP call
|
Example HTTP response
|
GetToken
Description
For a description of the GetToken
method, see the description of the Token
method in section 2.1 Token.
C# Syntax
ApiTokenModel GetToken(Guid ClientGuid, string UserName, string password,
int timeSpanInSeconds);
Parameters
The GetToken
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
ClientGuid |
ApiAuthenticationModel |
Client ID linked to a specific study in Viedoc. Can be obtained from Viedoc Admin. Required. |
UserName |
string |
Viedoc login username. Required. |
Password |
string |
Matching Viedoc login password. Required. |
TimeSpanInSeconds |
int |
Time (in seconds) that the authentication token will be valid for. Optional. Default is 300 seconds (5 min). Maximum is 1800 seconds (30 min). |
Returns
For a list of the returns of the GetToken
method, see the returns of the Token
method as described in section 2.1.4 Returns.
SubmitData
Description
The SubmitData
method can be used for submitting data into Viedoc.
C# Syntax
ApiSubmitResultModel SubmitData(string token, string odmXml,
ApiSubmitDataOptions options = null);
Parameters
The SubmitData
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
token |
string |
Authentication token. Can be obtained by invoking the Token method with client ID, username, and password. |
odmXml |
string |
The data to be uploaded in ODM format |
options |
ApiSubmitDataOptions |
Submit data options. Optional. See section 3.3 ApiSubmitDataOptions. |
Returns
The SubmitData
method returns an ApiSubmitResultModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Token |
string |
A new authentication token that can be used for authentication in subsequent requests |
Result |
ApiResultType |
Defines the type and status of the result returned from method invocation. See the section 3.2 ApiResultType for details. |
ErrorCode |
int |
In case of an error result: contains an integer specifying the type of error. See section 4 Error codes for details. |
ErrorMessage |
string |
In case of an error result: human readable description of the error |
TransactionGuid |
GUID |
A GUID assigned to the transaction that can be used to identify the transaction in future requests, for example when invoking TransactionStatus or TransactionData . Every single call to the SubmitData method will be assigned one transaction GUID, irrespective of how many subjects or data points are uploaded. |
Example call
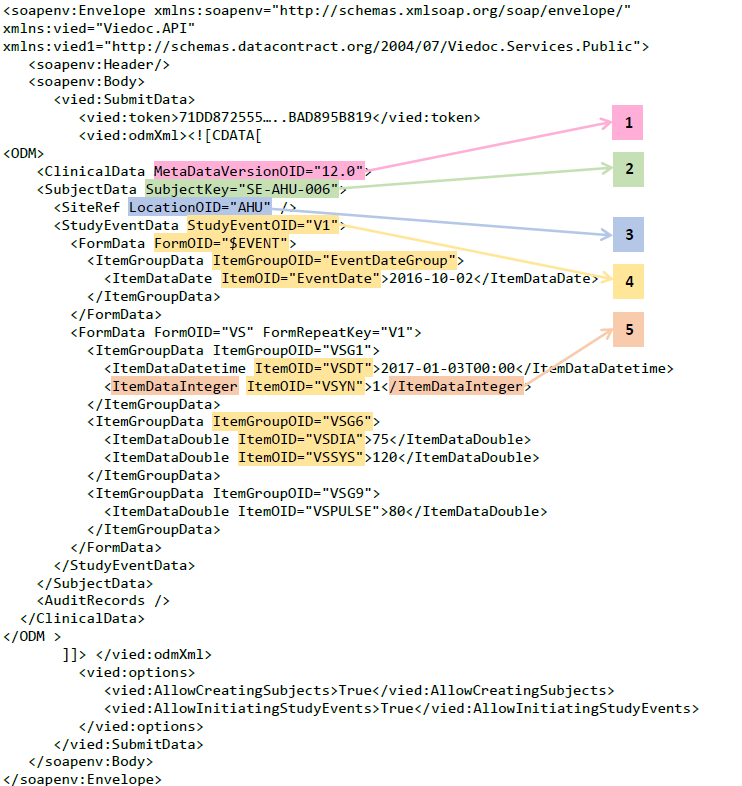
Note! To access the example call as a text that you can copy into your tool, click here.
Number | Item | Description |
---|---|---|
1 | MetaDataVersionOID |
[Version ]. [Revision ] of the metadata that will be used for the imported data |
2 | SubjectKey |
Subject key in Viedoc for the subject that the data will be imported to |
3 | LocationOID |
Study site ID, can be obtained from VIedoc Admin |
4 |
|
Event, form, or item Object Identifiers (OIDs), can be obtained from an exported metadata version or from Viedoc Designer Note! If the StudyEvent repeats, a StudyEventRepeatKey should be given. For example: |
5 | ItemDataInteger |
Allowed data value types are:
|
* CRF variables that collect time data have no container for time zone in Viedoc. Data in such variables is typically regarded to represent time in the same time zone as where the study site is located. Thus, it is recommended to submit time data without the time zone information, for example 2020-01-29T08:34:00. If time zone is of interest, for example if a blood sample was analyzed in a lab located in a different time zone, an additional CRF variable can be used to collect that information. When time zone information is submitted to Viedoc through the API (or the import application) as part of a data value, it will be factored into the data value. This is due to the fact that Viedoc has no place to store it. For example, 2000-01-01T00:00:00+01:00 (1 hour offset) will be converted to 1999-12-31T23:00:00Z (no offset) and will be visible in the CRF as 1999-12-31 23:00. For this reason, it is advisable to take care of any conversions required to get rid of time zone information before you submit time data to Viedoc.
Example response
|
Uploading an image file
It is possible to import a file, for example an image, to a File Upload item. This is similar to importing any other kind of data via the SubmitData
method. The file must be converted to a base64 string before it can be imported.
How the conversion is done depends on the programs that you are using, (for example, in Python you can use the b64encode function from the base64 module).
The item data type for the file upload item should be specified as ItemDataBase64Binary
in the ODM XML. In addition to its value (the base64 string), the v4:FileName property must be be specified. That is, the file name including the extension. The XML namespace v4 must be defined in the ODM start tag.
See the image below:
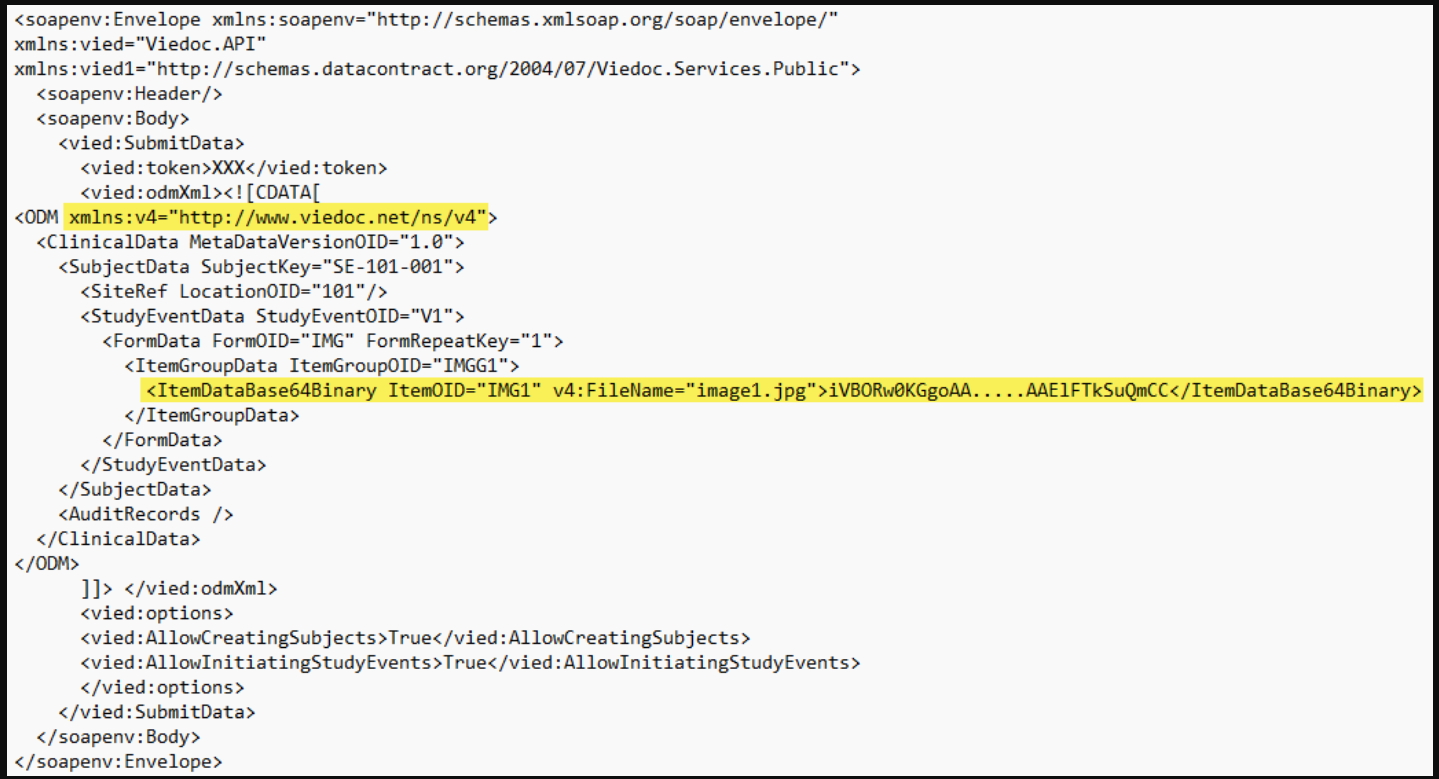
TransactionStatus
Description
The TransactionStatus
method can be used to check the import status of previously submitted data.
C# Syntax
ApiResultModel resultModel = TransactionStatus(string token, GUID transactionGUID);
Parameters
The TransactionStatus
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
token |
string |
Authentication token |
transactionGUID |
GUID |
The transaction GUID obtained when invoking the SubmitData method |
Returns
The TransactionStatus
method returns an ApiResultModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Token |
string |
A new authentication token that can be used for authentication in subsequent requests |
Result |
ApiResultType |
Defines the type and status of the result returned from method invocation. See section 3.2 ApiResultType for details. |
ErrorCode |
int |
In case of an error result: contains an integer specifying the type of error. See section 4 Error codes for details. |
ErrorMessage |
string |
In case of an error result: human readable description of the error |
Example call
|
Example response
|
TransactionData
Description
The TransactionData
method can be used to obtain previously submitted data.
C# Syntax
ApiTransactionDataModel dataModel = TransactionData(string token, GUID transactionGUID);
Parameters
The TransactionData
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
token |
string |
Authentication token |
transactionGUID |
GUID |
GUID obtained when invoking the SubmitData method |
Returns
The TransactionData
method returns an ApiTransactionDataModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Token |
string |
A new authentication token that can be used for authentication in subsequent requests |
Result |
ApiResultType |
Defines the type and status of the result returned from method invocation. See section 3.2 ApiResultType for details. |
ErrorCode |
int |
In case of an error result: contains an integer specifying the type of error. See section 4 Error codes for details. |
ErrorMessage |
string |
In case of an error result: human readable description of the error |
OdmXml |
string |
The uploaded data in ODM format |
Example call
|
Example response
|
GetMetaData
Description
The GetMetaData
method can be used to get any study metadata version in ODM format.
C# Syntax
ApiGetMetaDataResultModel metaDataResultModel = GetMetaData(string token, string metaDataOid, bool includeSdm, bool includeViedocExtensions);
Parameters
The GetMetaData
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
token |
string |
Authentication token |
metaDataOid |
string |
Metadata OID in the format: [Version]. [Revision]. For example, 1.1 means version 1 and revision 1. The metadata OID can be obtained from Viedoc Admin or Designer. |
includeSdm |
bool |
Defines whether Study Design Model (SDM) properties should be included in the exported metadata ODM file. Can be set to true or false , default is set to false . |
includeViedocExtensions |
bool |
Defines whether Viedoc-specific extension properties should be included in the exported metadata ODM file. Can be set to true or false , default is set to false . |
Returns
The GetMetaData
method returns an ApiGetMetaDataResultModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Token |
string |
A new authentication token that can be used for authentication in subsequent requests |
Result |
ApiResultType |
Defines the type and status of the result returned from method invocation. See section 3.2 ApiResultType for details. |
ErrorCode |
int |
In case of an error result: contains an integer specifying the type of error. See section 4 Error codes for details. |
ErrorMessage |
string |
In case of an error result: human readable description of the error |
OdmXml |
string |
ODM including the requested metadata version in the study |
Example call
|
Example response
|
GetMetaDataVersionForKeySets
Description
The GetMetaDataVersionForKeySets
method can be used to get the study design version(s) (metadata version) for a set of data point(s).
C# Syntax
ApiGetMetaDataVersionsResultModel GetMetaDataVersionsForKeySets(string token, List<ViedocKeySet> keySets)
Parameters
The GetMetaDataVersionForKeySets
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
token |
string |
Authentication token |
keySets |
List<ViedocKeySet> |
Contains a list of keysets for which study design (metadata) version should be fetched. All the individual keys in a keyset are optional and the returned study design version will be based on all the keys specified. See section 3.4 ViedocKeySet. |
Returns
The GetMetaDataVersionForKeySets
method returns an ApiGetMetaDataVersionsResultModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Token |
string |
A new authentication token that can be used for authentication in subsequent requests |
Result |
ApiResultType |
Defines the type and status of the result returned from method invocation. See section 3.2 ApiResultType for details. |
ErrorCode |
int |
In case of an error result: contains an integer specifying the type of error. See section 4 Error codes for details. |
ErrorMessage |
string |
In case of an error result: human readable description of the error |
KeySets |
List<ViedocKeySet> |
ODM including the requested metadata version in the study |
Example HTTP call
|
Example response
|
GetClinicalStudySites
Description
The GetClinicalStudySites
method returns information about the sites that a user has access to in Viedoc Clinic.
C# Syntax
ApiGetClinicalStudySitesResultModel GetClinicalStudySites(string token);
Parameters
The GetClinicalStudySites
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
token |
string |
Authentication token. |
Returns
The GetClinicalStudySites
method returns an ApiStudySiteModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Country |
string |
The country name |
CountryCode |
string |
Two-letter country code |
ExpectedNumberOfSubjectsEnrolled |
int |
The expected number of enrolled subjects on site |
ExpectedNumberOfSubjectsScreened |
int |
The expected number of screened subjects on site |
MaximumNumberOfSubjectsScreened |
int |
The maximum number of screened subjects on site |
Guid |
string |
Unique ID of the site |
SiteCode |
string |
Site code as set in Admin |
SiteName |
string |
Site name as set in Admin |
SiteNumber |
int |
Site number |
SiteType |
string |
Site type: Training or Production |
TimeZone |
string |
The Windows time zone ID |
TzOffset |
int |
The offset (in minutes) from UTC |
Example HTTP call
</soapenv:Envelope> |
Example HTTP response
|
GetClinicalData
Description
The GetClinicalData
method can be used for exporting clinical data in ODM format.
C# Syntax
ApiGetClinicalDataResultModel GetClinicalData(string token, ApiGetClinicalDataRequestModel options);
Parameters
The GetClinicalData
method has the following parameters:
Parameter | Data type | Description |
---|---|---|
token |
string |
Authentication token |
options |
ApiGetClinicalDataRequestModel |
Options and filters for clinical data export. See section 3.5 ApiGetClinicalDataRequestModel. |
Returns
The GetClinicalData
method returns an ApiGetClinicalDataResultModel
object that has the following properties:
Property | Data type | Description |
---|---|---|
Token |
string |
A new authentication token that can be used for authentication in subsequent requests |
Result |
ApiResultType |
Defines the type and status of the result returned from method invocation. An ApiResultType enum with the value Success or Error is used. |
ErrorCode |
int |
In case of an error result: contains an integer specifying the type of error. See section 4 Error codes for details. |
ErrorMessage |
string |
In case of an error result: human readable description of the error |
OdmXml |
string |
The exported data in ODM format |
Example HTTP call
Note! The order of the clauses is crucial. It is important to follow the order in the example code below.
|
Example HTTP response
MetaDataVersionOID="21.0">
v4:SiteSubjectSeqNo="6">
|
Note! GetClinicalData does not support StudyEventRepeatKey.
Complex Data Types
ApiAuthenticationModel
The ApiAuthenticationModel
data type contains the following elements:
Property | Data type | Description |
---|---|---|
ClientGUID |
GUID |
Client ID linked to a specific study in Viedoc. Can be obtained from Viedoc Admin. Required. |
UserName |
string |
Viedoc login username. Required. |
Password |
string |
Matching Viedoc login password. Required. |
TimeSpanInSeconds |
int |
Time (in seconds) that the authentication token will be valid for. Optional. Default is 300 seconds (5 min). Maximum is 1800 seconds (30 min). |
ApiResultType
ApiResultType
is an enum
data type with one of the following values*:
Pending |
The request is being processed and no result yet. |
Success |
The request has completed successfully. |
Error |
The request terminated with an error. See error code and message for a description of the error that occurred. |
InProgress |
Data import has started and date is currently being processed. |
PartialComplete |
Data import has started but is in an idle state waiting for remaining subjects to be unlocked so that data import can resume. |
Data import has started but a subject is not found due to invalid ID. The subject is not imported and the system continues to identify the next subject. | |
*For GetClinicalData , only an ApiResultType enum data type with the value Success or Error is used. |
ApiSubmitDataOptions
Property | Data type | Description |
---|---|---|
AllowCreatingSubjects |
bool |
Defines whether new subjects will be created during the data import when unmatched subjects are found. Can be set to true or false , default is set to true . |
AllowInitiatingStudyEvents |
bool |
Defines whether uninitiated events will be initiated during the data import. Can be set to true or false , default is true . |
ViedocKeySet
The ViedocKeySet
data type contains the following properties:
Property | Data type | Description |
---|---|---|
Uniqueld |
string |
For internal use only. The value of this property will be ignored if populated in a request. |
SubjectKey |
string |
Subject key of a subject in Viedoc |
StudySiteId |
int |
Database ID of the study site |
CountryCode |
string |
Two letter country code |
SiteCode |
string |
Site code as set in Admin. Required. |
SiteNo |
int |
Site number |
StudySujbectSeqNo |
int |
Sequence number of a subject on a study level |
SiteSubjectSeqNo |
int |
Sequence number of a subject on a site level |
StudyEventDefId |
string |
Study event OID as set in the study design |
StudyEventRepeatKey |
string |
Study event repeat key |
EventDate |
DateTime |
Event date in ISO8601 format |
FormDefId |
string |
Form OID as set in the study design |
FormRepeatKey |
string |
Form repeat key |
ItemDefId |
string |
Item OID as set in the study design |
MetaDataVersionOID |
string |
Study design OID (version) in the form [VERSION ]. [REVISION ]. Will be populated in the response based on the submitted values of all the previous keys. |
ApiGetClinicalDataRequestModel
The ApiGetClinicalDataRequestModel
data type contains the following properties:
Property | Data type | Description |
---|---|---|
SiteCode |
string |
Site code as set in Admin. Required. |
SubjectFilter |
string |
Subject filter using any string. Optional. |
SubjectKey |
string |
Subject key of a subject in Viedoc. Optional. |
StudyEventOID |
string |
Study event OID as set in the study design. Optional. |
FormOID |
string |
Form OID as set in the study design. Optional. |
ItemOID |
string |
Item OID as set in the study design. Optional. |
TimePeriodDateType |
ApiTimePeriodDateType |
SystemDate|EventDate. Optional. |
TimePeriodOption |
ApiTimePeriodOption |
Until|From|Between. Optional. |
FromDate |
DateTime |
Used to match data by entered or event date. Optional. |
ToDate |
DateTime |
Used to match data by entered or event date. Optional. |
ExcludeExtensions |
bool |
Defines whether to exclude the Study Design Model (SDM), Viedoc and audit trails. Can be set to true or false , default is set to false . |
IncludeAdminData |
bool |
Defines whether to include user and study site data in the export. Can be set to true or false , default is set tofalse . |
IncludeVisitDates |
bool |
Defines whether the event date form will be included in the export. The event date form includes the event date, planned date and the event window. Can be set to true or false , default is set to false . |
IncludeQueries |
bool |
Defines whether queries will be included in the export. Can be set to true or false , default is set to false . |
IncludeReviewStatus |
bool |
Defines whether review status will be included in the export. Can be set to true or false , default is set tofalse . |
IncludeSignatures |
bool |
Defines whether signatures will be included in the export. Can be set to true or false , default is set tofalse . |
IncludeMedicalCoding |
bool |
Defines whether medical coding will be included in the export. Can be set to true or false , default is set tofalse . |
IncludeSubjectStatus |
bool |
Defines whether to include the subject status in the export. Can be set to true or false , default is set to false . |
ViedocVersion |
string |
Defines which data structure version is used for the export. As of Viedoc release 4.39, the data structure version can be set to 4.38, 4.39 or Latest Viedoc Version. If nothing is specified, the Viedoc version set in the API configuration settings in Viedoc Admin is used. |
Error codes
The following table displays a list of error codes and their description.
Code | Message | Description |
---|---|---|
100 | Invalid username or password | The provided username or password is invalid. |
101 | Invalid Client GUID | The provided client ID is invalid. |
102 | Invalid token | The token is invalid. |
103 | NOT USED | |
104 | Xml data is required | No ODM XML data was included in the request. |
105 | NOT USED | |
106 | Invalid Client GUID/User | The provided token represents an invalid client GUID or an invalid user. This is very unlikely to occur when the token is generated from the system. |
107 | NOT USED | |
108 | NOT USED | |
109 | Unauthorized access, only user who submitted data can get transaction information |
TransactionData and TransactionStatus can only beinvoked by the user who submitted the data. |
110 | ||
111 | Permission denied | The user does not have access to the specified resource. |
112 | Metadata version not found | The requested metadata version could not be found in the study. |
114 | User is SSO user | The domain is set up for single sign-on, and API login is not supported. |
121 | Invalid study site | |
122 | User does not have export permission to site |
A workflow example
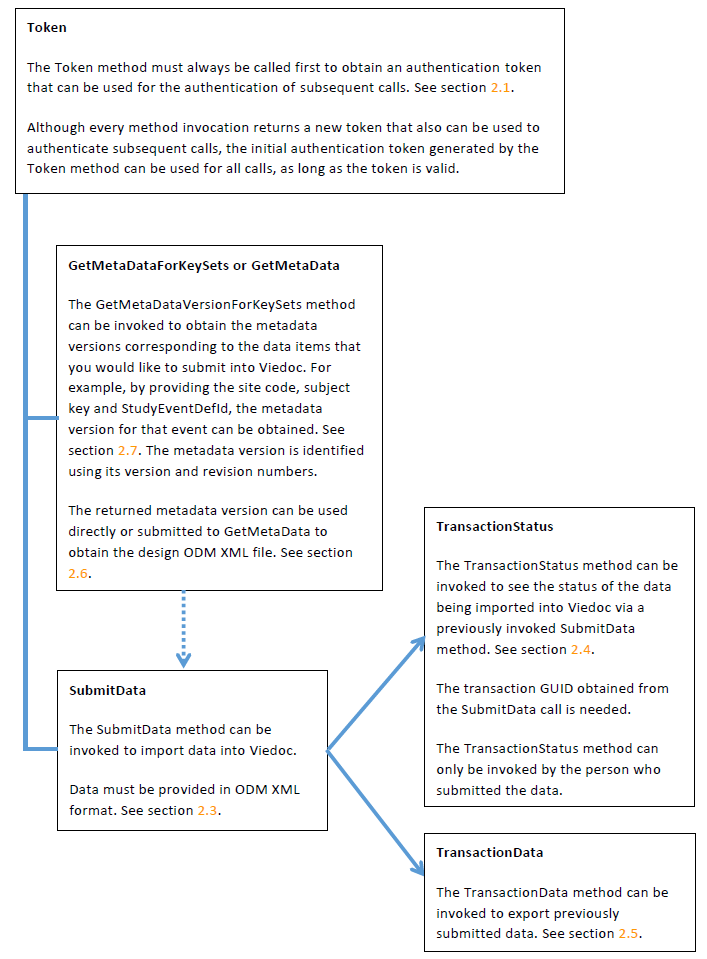
An example of how to submit data into Viedoc
Introduction
This chapter serves as an example of how to submit data into Viedoc. It provides instructions on where in Viedoc you can obtain the following information:
- A client ID
- Study site ID and design version
- Element OIDs
- Item data types
- Subject ID
This chapter also provides instructions to construct the clinical data file using the obtained information.
Obtaining the client ID
See API configuration.
Obtaining the study site code and design version
Note down the study site code and effective design version for the site or sites that data will be imported into. The
study site code and effective design are displayed in the study sites list in Viedoc Admin. The effective design version
is displayed in the form of [VERSION
].
[REVISION
] for each site separately.
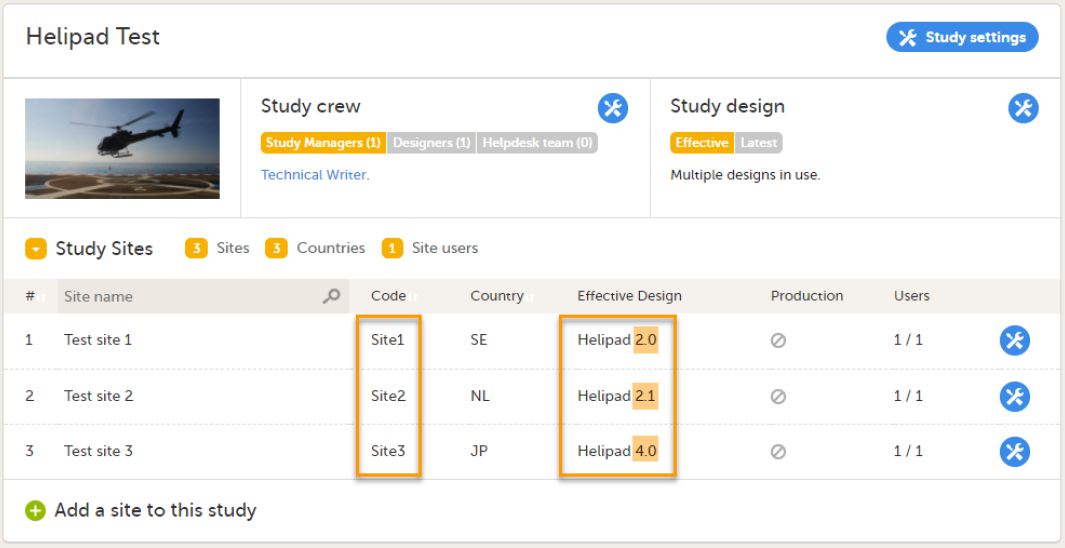
Obtaining the element OIDs
Obtain the following OIDs:
StudyEventOID
FormOID
ItemGroupOID
ItemOID
These OIDs can either be obtained from the study design in Viedoc Designer or by downloading the metadata
version by invoking the GetMetaData
API method.
|
If you choose to download the metadata version by invoking the GetMetaData
API method, search the returned ODM file for the following elements, and note down the OIDs:
StudyEventDef
(to obtain theStudyEventOID
)
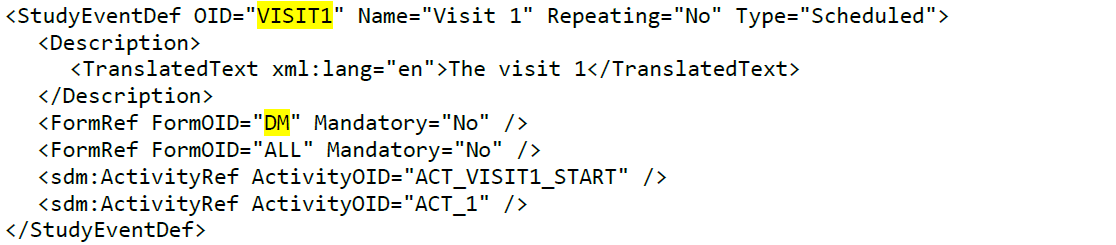
FormDef
(to obtain theFormOID
)

ItemGroupDef
(to obtain theItemGroupOID
) andItemRef
(to obtain theItemOID
)

Obtaining the item data types
Obtain the item data types. The item data types can be obtained from Viedoc Designer or found in the DataType
attribute of the ItemDef
element in ODM.
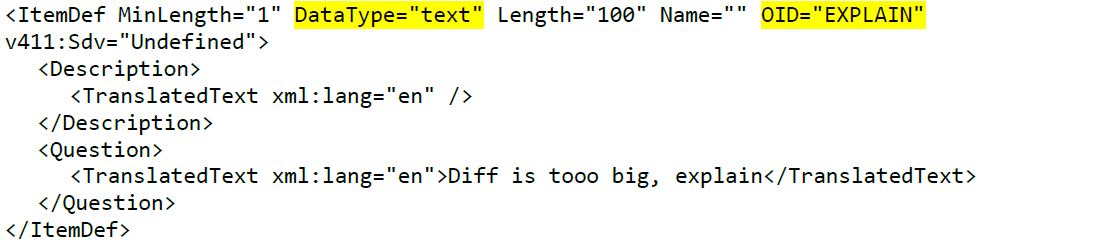
When constructing the ClinicalData
elements, use the data element corresponding to the item data type.
ItemDef Data type | ItemData Data type |
---|---|
String | ItemDataString |
Text | ItemDataString |
Integer | ItemDataInteger |
Double | ItemDataDouble |
DateTime | ItemDataDateTime |
Date | ItemDataDate |
Time | ItemDataTime |
Obtaining the subject key
The subject key is obtained from Viedoc Clinic.
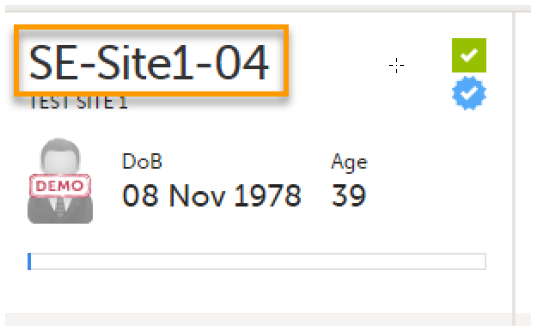
It is also possible to match subjects using the StudySubjectSeqNo
or the StudySiteSubjectSeqNo
. These are the sequence number of the subject in a study and study site respectively.
When trying to match data for an imported subject with a subject in Viedoc, the StudySubjectSeqNo
and StudySiteSubjectSeqNo
are used first. They can both be specified as extension attributes on the SubjectData
element in the ODM clinical data. If no matching subject is found using the StudySubjectSeqNo
or StudySiteSubjectSeqNo
, the subject key is used to find a matching subject.
If no matching subject could be found using either method, the following applies:
- If
AllowCreateSubjects
is set totrue
, a new subject is created. - If
AllowCreateSubjects
is set tofalse
, the subject is skipped.
TheDataImportLog
is indicated asPartialComplete
and shows which subject that does not exist.
When creating a new subject in Viedoc, the subject will receive the next available StudySubjectSeqNo
and StudySiteSubjectSeqNo
. These sequence numbers can be overridden in two different ways:
- By explicitly providing the subject sequence numbers as attributes.
- By including the subject sequence numbers in the subject key format, so that the subject sequence numbers can be extracted from the subject key. This requires the site code and site subject sequence number to be as specified in the Subject ID Generation Settings in the study design in Viedoc Designer.
Constructing the ODM XML ClinicalData file
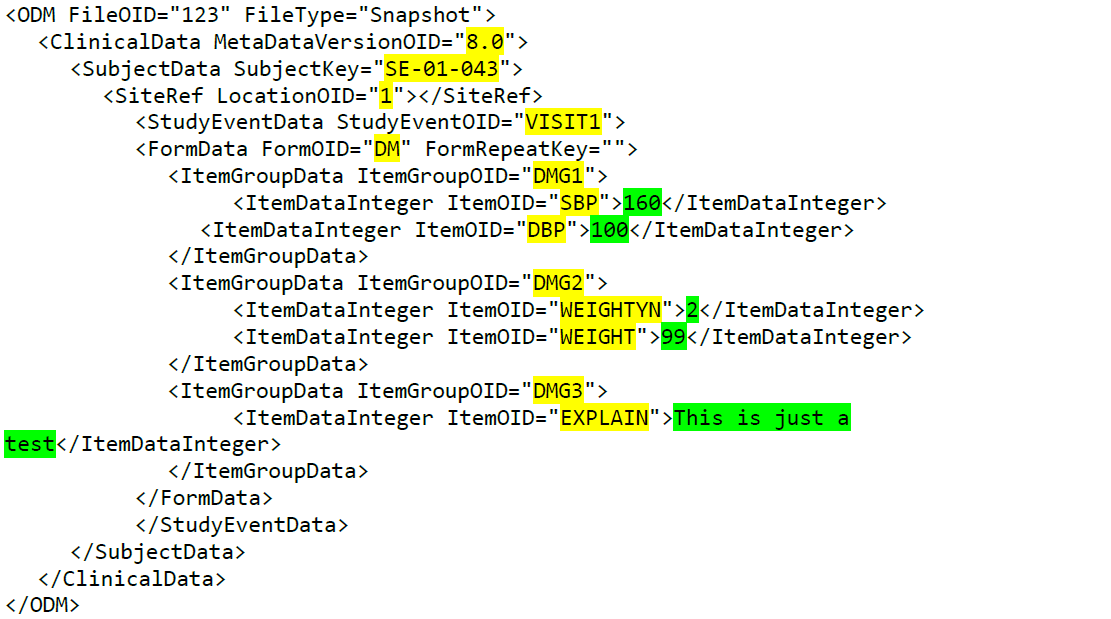
Note! To access the example ODM XML ClinicalData file as a text that you can copy into your tool, click here.
All text highlighted in yellow should be replaced with the MetaData version OID, Studysite OID, and Item OIDs obtained as previously described.
All text highlighted in green should be replaced with the values for the respective items.
The ClinicalData ODM can then be submitted using the SubmitData
method as described earlier, see section 2.3 SubmitData.